Armstrong number in shell script
Armstrong number is a integer number which is equal to the sum of of its own digits, each raised to the power of the number of digits in the original number.
For example 153 is a armstrong number, because Here we have the 3 digit count so my power will be 3. Now getting the sum of each digit raised to the power of the number of digits provides 153 that is equal to original number ( 13+53+33)=153
Armstrong number in shell script can be found by adding the the cube of each digit of the number
Given a number n by the user determine whether the given number is Armstrong number or not by calculating sum of each digits, each raised to power of number of digits.
Table of Contents
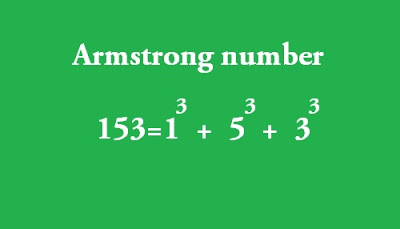
This program will check whether the number is armstrong or not using while and if else. if number is armstrong then it will display number is armstrong otherwise it will display number is not armstrong.
Shell script program for checking whether number is Armstrong or not
echo -n \”Enter the number: \”
read Number
Length=${#Number}
Sum=0
OldNumber=$Number
while [ $Number -ne 0 ]
do
Rem=$((Number%10))
Number=$((Number/10))
Power=$(echo \”$Rem ^ $Length\” | bc )
Sum=$((Sum+$Power))
done
if
[ $Sum -eq $OldNumber ]
then
echo \”$OldNumber is an Armstrong number\”
else
echo \”$OldNumber is not an Armstrong number\”
fi
Outcome Of Execution
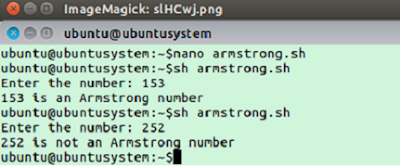
Extact Process of Identifying Armstrong Number
Still didn’t understand ?
Lets look the step by step process of getting or identifying the Armstrong number:
- Get a number and count the total number of digits in it.
- Separate the digits of the number.
- Do the each digit to the power of the total number of digits.
- Sum up all the results from the previous step
- Compare if the sum is equal to the original number.
- If the sum matches to the original number, that means then it is an Armstrong number otherwise not.
Applications of Armstrong Numbers
Armstrong number are used in various fields, especially in computer science and programming. they play around the number manipulation and understanding.
Here is the measure application of Armstrong number you must know:
Error detection: Armstrong numbers can be used for error detection in transmitting data. For instance, in the transmission process, if a number is distorted or manipulated then it’s no longer be an Armstrong number when it is received. that indicates a potential error.
Computer programming : For new programmer writing code to identify Armstrong numbers can be a fun and learning exercise for loop structures, conditionals, and basic arithmetic operations.
Digital Image Processing: Armstrong numbers are also used in image processing to create special effects on images. They help achieve certain patterns or distortions in an image by manipulating pixels.
FAQ About Finding ArmStrong number Shell Script?
What is an Armstrong number in shell script?
Armstrong number is a integer number that is equal to the sum of its own digits, each raised to the power of the number of digits in the original number.
What is an Example of Armstrong number?
153 is an Armstrong number because it equals the sum of its digits, each raised to the power of 3 (the number of digits in 153).( 13+53+33)=153